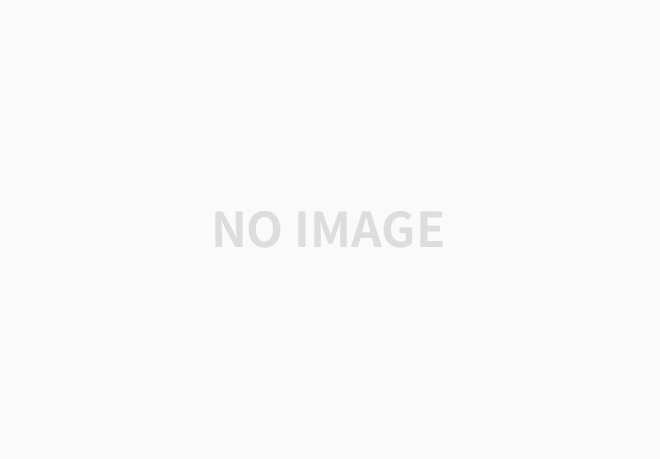
바닐라 JavaScript는 'use strict'를 사용하면 좀 더 체계적으로 코드를 짤 수 있다.
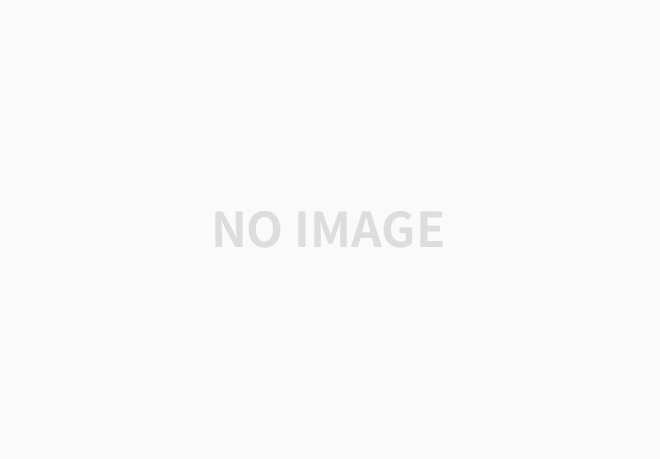
TypeScript(타입스크립트)는 JavaScript(자바스크립트) 위에 타입이 올라간 것이기 때문에, 위와 같은 것을 생각해두면 좋다.
그리고 JS를 배우고 나면 TypeScript를 금방 배울수 있다.
JS관련 문법
1. 변수형, 문자열 출력 표기 및 연산자
// 문법
//let : 변수를 선언할때 사용 ES6
let name='evan';
console.log(name);
name = 'ggg';
console.log(name);
//var hoisting이란 어디에서 선언을했던 선언을 끌어올려줌
age=4;
var age;//이런식으로 위에서 먼저 써버리는게가능
//var는 {블록}에서 상관 안함 문제발생.
{
age=4;
}
console.log(age);
//Constants 상수 : 1.보안상 2.스레드안정성 3.실수절감
const dayInweek = 7;
const maxNumber = 5;
//변수타입 function, firs-class function
//js는 숫자타입 상관없이 number타입
const count =15;
const a=1;
const infinity = 1/0;
const negativeInfinity = -1/0;
const nAn = 'not a number' /2;
const char = 'c';
const brend = 'brenden';
const greeting = 'hi' + brend;
// 백태그 ` 를 이용하면 이렇게 띄어쓰기까지 다 인식
console.log(`value: ${char}, type: ${typeof greeting}` );
console.log('value: ' + char + ', type:' + typeof greeting);
const bool = 3<1; // false
//심볼을 만들때 사용
const sym1 = Symbol('id');
const sym2 = Symbol('id');
console.log(sym1 === sym2); //flase. 심볼은 동일하게 작성해도 다르게 나옴 flase
const gSym1 = Symbol.for('id');//주어진 string에 맞는 심볼을 만들어줘
const gSym2 = Symbol.for('id');//주어진 string에 맞는 심볼을 만들어줘
console.log(gSym1 === gSym2); // true
//object, real-life
const ellie = {name = 'ellie', age:20};
ellie.age = 21;
//Dynamic typing : 동적타입(프로그램이 동작할때 변경)
let text = 'hello';
console.log(text.charAt(0));//h출력
console.log(`val: ${text}, type: ${typeof text}`);
text = 1;
console.log(`val: ${text}, type: ${typeof text}`);
text = '7' + 5;
console.log(`val: ${text}, type: ${typeof text}`);
text = '8' / '2';
console.log(text.charAt(0));//에러~~
console.log(`val: ${text}, type: ${typeof text}`);
- 가능하다면 안정성, 보안성을 위하여 한번 변경하지 않을 변수는 const를 사용하는것이 좋다.
- Immutabel Data : primitive 변수타입은 데이터 수정은 변경 불가. 완전 새로 선언만 가능
- Mutable Data : 모든 수정가능한 오브젝트. in JS
2. 연산자, 조건문, 반복문
//1. String concatenation
console.log('my' + 'cat');
console.log('1' + 2);
console.log(`string literals :
백슬러쉬
1 +2 = ${1 + 2}`);
//2. Numeric operators
console.log(1 + 1);
console.log(2 ** 3); //지수
//3. 증감연산자
let counter = 2;
const preIncrement = ++counter;
console.log(`preIncrement : ${preIncrement}`);
//4. Assignment operators
let x= 3;
let y =4;
x+=y;
//5. 비교연산자
console.log(10 < 6); //less than
//6. 논리연산자(or|| and&& not!)
const value1 = false;
const value2 = 4<2;//false
function check(){
for(let i=0; i<10; i++){
//wasting time
console.log('😊');
}
return true;
}
//7.Equality
const stringFive = '5';
const numberFive = 5;
console.log(stringFive == numberFive);//true 타입달라도
//string Equality 검사 (타입도 같아야됨)
console.log(stringFive === numberFive);//false
console.log(stringFive !== numberFive);//true
console.log(0==false);//true 약한검사는 통과
console.log(0===false)//false 다른타입.
console.log(null == undefined);//true
console.log(null === undefined);//false 다른타입
//8. 조건문 if else
const name = 'evan';
if(name === 'evan'){
console.log('hello world');
}else if(name === 'code'){
}else{
}
//9. Ternary operator : ?
console.log(name === 'evan' ? 'yes' : 'no');
//10. switch
switch(name){
case 'evan':
console.log('evan hello');
break;
case 'code':
console('hi code');
default:
//머시기
}
//11. 반복문
let i =3;
while(i>0){
}
do{
//선실행
}while(i>5){
//머시기
}
for(let k=5; k>3; k--){
console.log('for문입니다' + k);
}
3. Function : 먼저 선언되므로 아래에서 선언해도 먼저 인식해놓는다.
타입스크립트는 이처럼 매개변수의 형과 리턴값의 형을 정해주어야한다.
function log(message : string) : number{
console.log(message);
return 0;
}
하지만 자바스크립트는 따로 형을 정해주지않기 때문에 아래와 같이 표현한다.
function log(message){
console.log(message);
return 0;
}
디폴트 매개변수
function showMessage(One, Two='없으면 출력되는값'){
console.log(One , Two);
}
showMessage('Hi');
배열출력
function printAll(...args){//배열 매개변수
//1번째 출력방법
for(let i =0; i<args.length; i++){
console.log(args[i]);
}
//2번째 출력방법
for(const arg of args){
console.log(arg);
}
//3번째 출력방법
args.forEach((arg) => console.log(arg));
}
printAll('dream' , 'coding', 'skmouse');
빠른 리턴, 빠른 탈출
//Early return, early exit
function upgradeUser(user){
if(user.point > 10){
//내용
}
}
function upgradeUser(user){
if(user.point <= 10){
return;
}
//내용
}
Annonymous function
const print = function(){//anonymous function
console.log('print');
};
print();
=> Arrow Function : 함수 간결하게
const simplePrint = () => console.log('hi');
const add = (a,b) => a + b;
콜백함수 : 자바스크립트는 이벤트 기반 언어이기 때문이다. 자바스크립트는 다음 명령어를 실행하기 전 이전 명령어의 응답을 기다리기보단, 다른 이벤트들을 기다리며 계속 명령을 수행한다.
//콜백 function
function randomQuiz(answer, printYes, printNo){
if(answer === 'love you'){
printYes();
}else{
printNo();
}
}
const printYes = function(){
console.log('yes');
}
const printNo = function print(){//print쓰는거는 디버깅할때 함수이름 나오게하려고 사용
console.log('no');
print();//재귀함수(이건 무한루프긴함)
}
randomQuiz('wrong', printYes, printNo);
지연시간이 소요되는경우를 생각해보자.
function doHomework(subject, callback) {
alert(`Starting my ${subject} homework.`);
callback();
}
function alertFinished(){
alert('Finished my homework');
}
doHomework('math', alertFinished);
[번역] JavaScript: 도대체 콜백이 뭔데?
이 문서는 Brandon Morelli의 JavaScript: What the heck is a Callback? 을 번역한 것입니다. 잘못된 부분이 있는 경우 알려주시면 감사하겠습니다.
medium.com
'웹(Web) > 프론트엔드(Frontend)' 카테고리의 다른 글
JavaScript 정리3(Object, 프로퍼티 전달, 배열 출력, clone) (0) | 2021.03.15 |
---|---|
JavaScript 정리2(클래스, 상속, 다양성) (0) | 2021.03.15 |
모션그래픽 p5.js(Processing 같은원리) (1) | 2021.03.01 |
CSS 정리(선택자, Flexbox, 속성값 등) (0) | 2021.02.21 |
css 색 디자인color tool (0) | 2021.02.21 |